Practical 1
Contents
Practical 1#
Getting started with Python programming#
Aim#
The aim of this practical is to learn the Python programming language syntax and its core programming concepts and how to use them. It is the first step in learning Python programming.
Objectives#
During this practical you will learn how to:
create a Python program (.py file)
create a Jupyter notebook (.ipynb file)
define variables
use different data types in Python
use different data structures in Python and perform different operations with them
how to call functions
use different control flow statements: if statement, for and while loops to control flow of code execution
how to find help
Instructions#
The best way to do this practical is to read these notes which will introduce you to the core concepts of Python programming. These notes are designed with all the details you would need to be able to perform the exercises in this practical. In general, you would not need to refer to additional texts and resources as these notes have been explained in detail with accompanying visual aids and examples to explain concepts to beginners. Apart from the exercises listed below, there are also examples throughout the notes which provide insight on the concepts introduced, and therefore, it is recommended that you also run these examples as you progress through the practical notes.
If you are an experienced Python programmer, you might find that you already know about the content in these notes. You may want to skip sections of notes that you already know and attempt the exercises that are at the higher levels.
Exercises levels#
Exercises in this practical are labelled with the level of difficulty of the respective exercise:
Level |
Description |
---|---|
|
Level 1: Excercises in Level 1 are simple excersises designed to get you familiar with the Python syntax. If you already know how to program in Python, you may skip these exercises. |
|
Level 2: Excercises in Level 2 combine different Python programming concepts to solve simple problems. |
|
Level 3: Exercises in Level 3 test not just the understanding of the Python syntax but also how Python can be used to solve problems. |
Exercises#
This practical is composed of the following exercises.
Exercise |
Description |
Level |
---|---|---|
Markdown in Jupyter Notebook: This exercise exposes us to Markdown in Jupyter Notebooks. |
|
|
More text formatting in Markdown: This exercise uses more formatting options in our Markdown Cells. |
|
|
Arithmetic operators: In this exercise we try the different arithmetic operators. |
|
|
Variables and their types: In this exercise we explore how values and types of variables change as we apply operations on them. |
|
|
Lists: This exercise explores lists and other operations we can do on them. |
|
|
Modifying Lists: In this exercise different list methods are called to explore how we can do modifications on lists. |
|
|
Joining strings: In this exercise we learn how to join a list of words into a string. |
|
|
Splitting strings: This exercise counts the number of words in a sentence. |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Fibonacci sequence: This exercise creates a program that writes a Fibonacci sequence. |
|
In the programming world, many organisations award badges to learners on the acquisition of a new skill or completion of a milestone. If you attempted and solved all the exercises of this practical, you definitely deserve your first badge of this course.
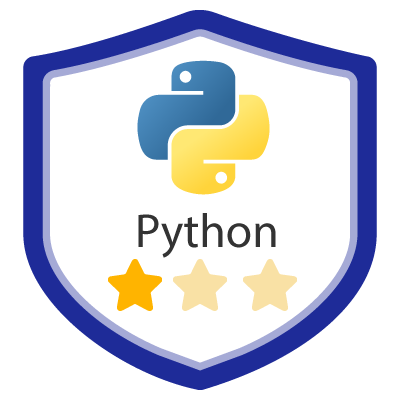