Arithmetic Operations
Contents
1.5. Arithmetic Operations#
Below are some of the most used mathematical operations.
Operator/Function |
Description |
Example |
---|---|---|
+ |
Addition |
1 + 2 returns 3 |
- |
Subtraction |
3 - 1 returns 2 |
* |
Multiplication |
3 * 4 returns 12 |
/ |
Division |
4/2 returns 2.0 |
// |
Floor division: returns the integer part of a division result (without the fraction part) |
5//3 returns 1 |
% |
Remainder |
5 % 2 returns 1 |
** |
to the power of |
3 ** 2 returns 9 |
|
x to the power of y (same as **) |
|
|
absolute value of x |
|
|
rounds x to n decimal places |
|
Python follows the traditional mathematical rules of precedence (BODMAS). You can find more about these rules here.
Exercise 1.3 (Arithmetic Operators)
Level:
a. Try the examples in Table 1.1 in a jupyter notebook code cells.
b. Calculate: \(8 + (10 + 4^3 + 2)\)
1.6. Variables#
The next sections of this practical are going to focus on the python code. Try all the python code in these sections in the Jupyter Notebook code cell and run each cell to see the output.
If we want to write some useful code, we need to learn how to create variables.
Programming concept
A variable (also known as object reference) is a reference name that points to a value in memory. A variable can be declared as following:
variable = value
Let us create our first variable:
weight_kg = 55
When executing this, Python creates an int
object in memory with the value of 55
in it and creates a variable
weight_kg
that points to it. Thus, in this example, the variable weight_kg
has been assigned the value of 55
as
shown in Fig. 1.3.
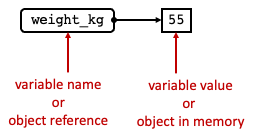
Fig. 1.3 Representation of a variable in memory.#
When assigning a value to a variable, the Python interpreter does not print the value back. To print the value of the
variable you need to use the print()
function (e.g., print(weight_kg)
).
1.6.1. Variable naming conventions#
Variables can be given any name, however it is important to follow the variable naming conventions in Python. These conventions are in place as guidelines so that there is consistence in the way we write code. A few rules to keep in mind when naming variables are:
Variables are case-sensitive (e.g.,
weight
andWeight
are two different variables).Variables should be lowercase, with words separated by underscores e.g.,
weight_kg
Do not start variables with numbers.
Use only letters (underscores and digits if necessary).
Variables should be descriptive to improve readability e.g.,
asdjks
is not a good variable name.Variables should not be too long e.g.,
this_is_my_weight_in_kg
is not a good variable name.Do not use function names, class names, data types or other keywords as variable names.
Detailed guidelines on Python naming conventions can be found in the PEP 8; the de facto code style guide for Python and Google Python Style Guide.