More on strings and functions
Contents
1.11. More on strings and functions#
1.11.1. String indexing and slicing#
We have already looked briefly at strings previously. Like tuples and lists, strings too are of Sequence type in Python, therefore they support slicing, membership operators, concatenation, replication and the other utility functions we mentioned in the Tuples section. Instead of data items, however, strings are composed of a sequence of characters. Fig. 1.9 below shows an example of the sequence of characters in the string “Hello World!” together with its index positions.

Fig. 1.9 Indexes for str “Hello World!#
Thus, we can extract characters from a string as in the code below:
And we can slice a string as below:
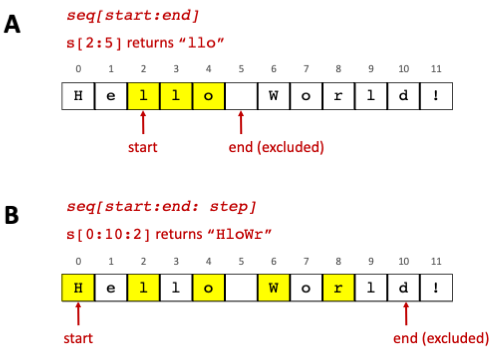
Fig. 1.10 String slicing.#
1.11.2. Strings are immutable#
Like tuples, since strings are immutable, one cannot change the contents of a character in a string. Trying to modify a character in a string will throw an error as shown below:
1.11.3. String utility functions and methods#
More useful functions and methods for strings are listed in Table 1.7. A list of all the String methods in Python can be found here.
Function |
Description |
---|---|
|
returns the length of the string |
|
returns |
|
returns |
|
returns |
|
returns the number of times |
Exercise 1.7 (Joining strings)
Level:
Given a list of str
objects defined as below:
words = ["keep", "calm", "and", "carry", "on"]
Join all the str
objects together and separate them by a space into one str
object and print it.
The final string should have the following value: “keep calm and carry on”
Tip
Use the join()
String method. Look at its documentation
to see how this can be used.
Exercise 1.8 (Spliting strings)
Level:
In this exercise write code that counts and prints the number of words in the famous quote from Star Wars: “May the Force be with you”